Java linked programming, Homework Help
Try to change a list of characters(char) in linked list from lower case to upper case.
heres my code:
// Main program
package ll_csc300_sp2016;
public class Ll_csc300_sp2016
{
public static void main(String[] args)
{
List myList = new List();
myList.insertAtHead(‘a’); //list input
myList.insertAtHead(‘b’);
myList.insertAtHead(‘c’);
myList.insertAtHead(‘d’);
myList.insertAtHead(‘e’);
myList.display();
myList.toUppercase();
}
}
// Class List
/*
list manager class.
*/
package ll_csc300_sp2016;
public class List
{
public Node head; //list manager
List () { head = null; }
List (Node n) { head = n; }
public void displayIterative()
{
Node p = head;
while (p != null)
{
System.out.println(p.input); // if its not empty go to the next one
p = p.next;
}
}
public void display()
{
displayR(head); //display the file
}
private void displayR(Node p)
{
if (p == null) return;
System.out.println(p.input);
displayR(p.next);
}
public Node find (char a)
{
Node p = head;
while (p != null)
{
if (p.input == a)
return p;
p = p.next;
}
return null;
}
public Node toUppercase (char a)
{
Node p = head;
while (p != null)
{
if (p.input == ‘a’) // if input is lowercase char
a = Character.toUpperCase(a); // change it to uppercase
p = p.next;
return p;
//p = p.next;
//return p;
}
return null;
}
public void insertAtHead(char i) //input
{
Node newOne = new Node(i);
newOne.next = head;
head = newOne;
}
}
//class node
/*
node class
*/
package ll_csc300_sp2016;
public class Node
{
public char input;
public Node next;
Node() //constructor
{
next = null;
}
Node(char d)
{
input = d;
next = null;
}
}
"Looking for a Similar Assignment? Order now and Get 10% Discount! Use Code "GET10" in your order"
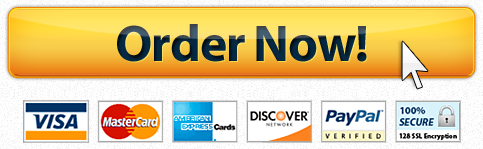
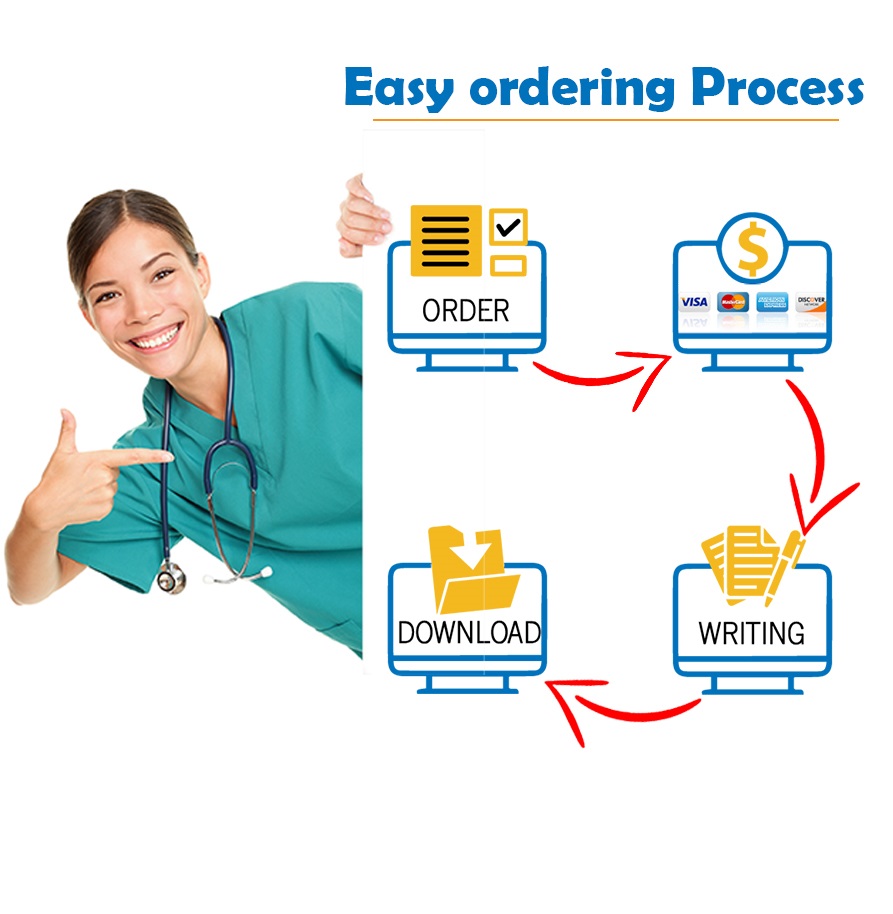